HTML & CSS Week 3 - Using the div tag to manage your layout
We've got some basic elements of a web page now. But your page doesn't look quite like the other pages out there. It just starts in the top left corner, and runs across the page. It doesn't have any visual structure. it doesn't have any layout. How might we change that?
The div tag
The <div> tag is short for division, in the sense that you've divided your content into sections. And that's all it does, really. It marks out a bit of your page as a section. But that is very useful indeed, because we can do a whole bunch of things with those sections.
Open your index.html file from last week, and we'll give it a bit of structure. For a start, let's give it a title bar. Right at the top of your page, just after the <body> tag, add a div. And we're going to give it an attribute, one that we haven't seen before - id. Id is like a name for yor tag, and lets you refer to it individually. Each id has to be unique on your page. We'll call our div 'header'.
We can use the id to apply a style to just that div, and the way we do that is by putting a # in front of its name. So #header means "the tag with the id='header' attribute". So add a style for it to so that we can see it. Your code should look like this:
<style>
#header {
color:white;
background-color:rgb(99, 4, 77);
height: 200px;
}
</style>
<div id='header'>This is my header. I can put anything I like in it.</div>
Depending what else you've got on your page, you should now have something a bit like this: a coloured header bar going across the entire top of the page.
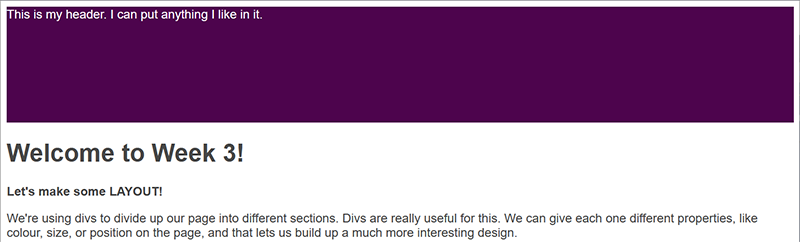
Now, I like that it goes all the way along, but notice how it doesn't go quite to the edges? That's because of another style,
called padding. Padding is the space between a container and its content. And this is either the body's padding, or its margin.
margin put space around an element, while paddingputs a gap between an element and everything inside it. We didn't
put these styles there - the browser did. It's called a default style. But we can switch them off, by setting both to 0
in the body style:
body {
margin: 0;
padding: 0;
}
What else do I not like? Well, the font in the header looks a bit small for a title. And text does look a bit weird when it goes right to the edge like that, so I can add some padding to that. And when you do that, you might notice that the div gets bigger, because the padding gets added to the height you already set. So I'll make the height a smaller again.
#header {
color:white;
background-color:rgb(99, 4, 77);
height: 110px;
font-size:28px;
padding:20px;
}
Another div
I'll tell you what else I don't like: loooong lines of text that go across the whole page. They're hard to read - there's a reason that magazines break up text into short columns. So I'm going to make a div for all of my content, and give it a width that's a bit more manageable
<style>
#content {
background-color:white;
width: 800px;
margin-left:auto;
margin-right:auto;
}
</style>
<div id='content'>
Everything else in my page is going to go between these div tags
</div>
What I've done here is a little trick to centre the div. I've given it a margin on it's left and right side. I've used a special value, auto, but it doesn't actually matter what I use: as long as the values are the same, the browser thinks, "Well I can't make it be x pixels from the left AND x pixels from the right, so I'll just put it in the middle."
To see this in action, we can change the body colour while keeping the content div white.
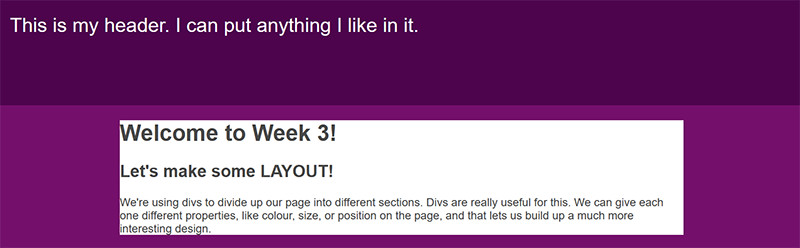
Hmmm... Maybe I quite like that. But now I can see that there's a gap above my content div. The reason for that is weird and complicated, but I can get rid of it, and make it all look nicer, by giving my div some padding:
padding: 10px 16px;
A note on 'shorthand'
Some CSS properties can be written in a shorthand form, and padding, border and margin are examples of this. Each one is actually 4 different properties: eg border-top, border-right, border-bottom, border-left, but to keep things neat we can specify all of those values in a single declaration, as shown below.
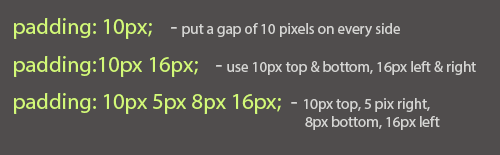
What next?
You've got quite a few tools to play with now. Try building up your web page with some more content, and adding extra divs to format and organise it. There's no limit to what you can do, so if you've got an idea, ask and we'll try to explain how to do it.
The page I wrote
You can look at the page I just made, and use 'View Source' to copy the code if you get stuck.
It's here!