Python Cheat Sheet - Week 4
A bit of fun, this week, mostly using the things you've learned in the previous three. That, and your own creativity! But there are also some new drawing concepts, so still plenty to get your head around.
Code is shown in blue.
Colours
The lesson begins with a welcome explanation of colour (there's more here). It will take a while to get used to RGB colours, so why not open the W3 Schools' colour picker in a new tab? That way you can use it every time you need a new colour. This tool is designed for the web, where hex is default, but in Python, you don't need the 'rgb' bit, just the numbers.
Of course, you don't need a colour picker - you can just pick 3 numbers at random, as long as none is larger than 255.
Later in the tutorial you'll see how you can add a fourth number to this, to set the opacity - how much you can see through a colour to whatever is drawn beneath.
The Grid
That grid() function - what's that about? Who knows. Look at the top of the code and you'll see it's a library that's being imported. To turn it off, just put a # in front, turning it into a comment.
Get drawing!
So what do you need to do? Handily, there's a quick rundown of the shape drawing functions in the instructions. And then for many of the shapes you draw, you'll want to specify an outline and fill colour, or even turn them off:
stroke(0, 0, 0)
stroke_weight(5)
no_stroke()
fill(255, 255, 140)
no_fill()
Pro Tip: If you temporarily turn off the fill, it might help you to see where the shapes are being positioned underneath other ones.
Calculating position
Most faces have roughly symmetrical features, so assuming yours is centred on the page, you may want to line things up a certain distance either side of that centre point. Let's say your head is a circle, centred in an area 400 x 400, you probably want eyes of the same size, the same distance from the centre. The first trick is to use a variable for the eye size, that way, when you want to change it, you only have to change it in one place. The next trick is to use maths for the x-position. So you could say, I want the eyes 60px away from the centre, so that's 200-60=140 for one, and 200+60=260 for the other. Or you could let the code do the maths for you:
eye_size = 30
ellipse(width/2 - 60, 180, eye_size, eye_size)
ellipse(width/2 + 60, 180, eye_size, eye_size)
Improve readability with functions
If all our code goes in the draw() function, it's quickly going to get messy. We can improve this by making functions for different parts of the drawing. This is especially good where the code gets used more than once - drawing eyes, for example. We could call the eye() function twice, and pass it a variable each time to describe where on the x-axis to draw it:
eye(140)
eye(260)
Or we could use translate(). Translate moves the screen, so that if we draw in, say, the centre of it, that centre is no longer in the middle. We can also use push_matrix() to store where the screen was before we started messing about with it, and pop_matrix() to put it back there again before we draw anything else:
push_matrix()
translate(-60, 0)
eye()
translate(120, 0)
eye()
pop_matrix()
Translate() moves the screen, but you can also rotate() it. This may seem a slightly confusing way of doing things, but that's because we're using p5, which emulates a language called 'Processing'. So it's not really the way Python works; it's the way Processing/p5 works
The Result
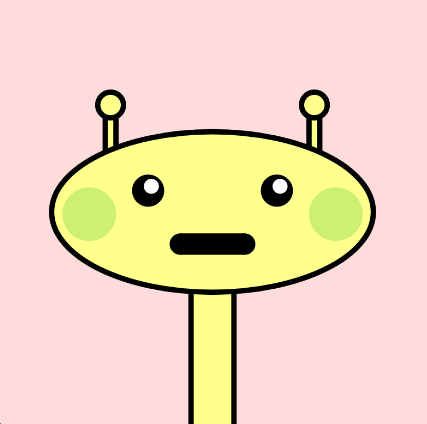
The Code
Here's the code for the odd chap I created when going through the tutorial. Feel free to copy it into your editor and play around with it.
#!/bin/python3
from p5 import *
from grid import *
def setup():
# Put code to run once here
size(400, 400) # width and height
def draw():
# Put code to run every frame here
background(255, 220, 220)
# Add code to draw your face here
#grid() # add a # to the beginning of this line to hide the grid
# Neck
stroke_weight(5)
stroke(0, 0, 0)
fill(255, 255, 140)
rect(180, 220, 40, 200)
# Antenna
rect(100, 100, 10, 50)
rect(290, 100, 10, 50)
ellipse(105, 100, 24, 24)
ellipse(295, 100, 24, 24)
# Head
ellipse(width/2, height/2, 300, 150)
# Eyes
push_matrix()
translate(-60, 0)
eye()
translate(120, 0)
eye()
pop_matrix()
# Mouth
no_stroke()
fill(0, 0, 0)
rect(width/2-30, 220, 60, 20)
ellipse(width/2-30, 230, 20, 20)
ellipse(width/2+30, 230, 20, 20)
# Cheeks
fill(0, 180, 0, 50)
ellipse(width/2+115, 202, 50, 50)
ellipse(width/2-115, 202, 50, 50)
def eye():
fill(0, 0, 0)
eye_size = 30
no_stroke()
ellipse(width/2, 180, eye_size, eye_size)
fill(255, 255, 255)
pup_size = 14
ellipse(width/2+3, 176, pup_size, pup_size)
# Keep this to run your code
run()